mirror of
https://github.com/lalbornoz/roar.git
synced 2024-11-02 13:56:39 +00:00
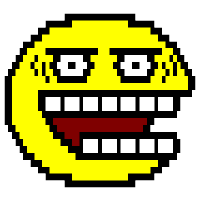
1) Assets window: adds clear list context menu item. 2) Assets window: allow deleting multiple selected items. 3) Assets window: fix list view cursor key navigation. 4) Backend: correctly blend transparent background cursor cells with canvas character cells. 5) Backend: correctly determine cell size & set font size. 6) Backend: correctly unmask cursor. 7) Backend: disable font anti-aliasing on Windows. 8) Backend: render transparent background cells as RGBA #303030FF. 9) Canvas window: adds <F1> accelerator for `View melp?' menu item. 10) Canvas window: implement {dockable,floating} toolbars w/ wx' AUI framework. 11) Canvas window: separate tools toolbar from edit commands toolbar & dock both on right-hand side alongside each other. 12) Flip horizontally tool: flip characters, including some Unicode symbols. 13) Flip vertically tool: flip additional Unicode symbols. 14) Text tool: don't process keyboard events w/ either of <{Alt,AltGr,Ctrl}> modifiers. assets/images/roar.png: updated.
32 lines
1007 B
Python
32 lines
1007 B
Python
#!/usr/bin/env python3
|
||
#
|
||
# OperatorFlipHorizontal.py
|
||
# Copyright (c) 2018, 2019 Lucio Andrés Illanes Albornoz <lucio@lucioillanes.de>
|
||
#
|
||
|
||
from Operator import Operator
|
||
|
||
# TODO <https://en.wikipedia.org/wiki/Box_Drawing_(Unicode_block)>
|
||
|
||
class OperatorFlipHorizontal(Operator):
|
||
name = "Flip horizontally"
|
||
flipPairs = {
|
||
"/":"\\", "╱":"╲",
|
||
"▀":"▄", "▁":"▔", "▖":"▘", "▗":"▝",
|
||
"▙":"▛", "▚":"▞", "▜":"▟",
|
||
}
|
||
|
||
def apply(self, region):
|
||
region.reverse()
|
||
for numRow in range(len(region)):
|
||
for numCol in range(len(region[numRow])):
|
||
if region[numRow][numCol][3] in self.flipPairs:
|
||
region[numRow][numCol][3] = self.flipPairs[region[numRow][numCol][3]]
|
||
return region
|
||
|
||
def __init__(self, *args):
|
||
for flipPairKey in list(self.flipPairs.keys()):
|
||
self.flipPairs[self.flipPairs[flipPairKey]] = flipPairKey
|
||
|
||
# vim:expandtab foldmethod=marker sw=4 ts=4 tw=120
|